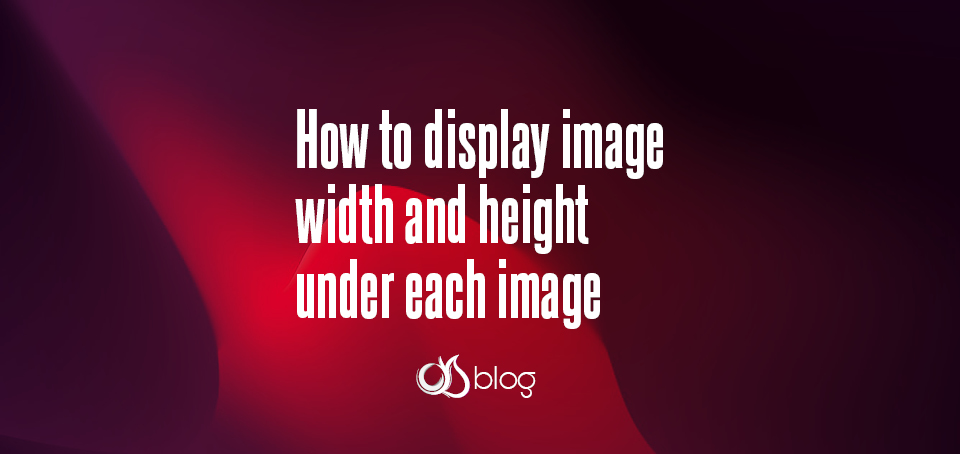
The code display the width and height on your website
To display the size (i.e. display the width and height) of an image on a webpage using HTML, you can use JavaScript to dynamically fetch the dimensions of the image and update the HTML accordingly. Here’s an example:
<!DOCTYPE html>
<html>
<head>
<script>
function showImageSize() {
var img = document.getElementById('myImage');
var width = img.clientWidth;
var height = img.clientHeight;
var sizeText = width + 'px x ' + height + 'px';
var sizeElement = document.getElementById('imageSize');
sizeElement.textContent = sizeText;
}
</script>
</head>
<body>
<img id="myImage" src="path/to/your/image.jpg" onload="showImageSize()">
<p>Image Size: <span id="imageSize"></span></p>
</body>
</html>
How it works
In this example, the showImageSize
function is called when the image is loaded. It retrieves the image element using its id
, myImage
, and then fetches the width and height of the image using the clientWidth
and clientHeight
properties respectively. The calculated dimensions are then assigned to the textContent
property of the imageSize
element, which is initially empty.
Make sure to replace 'path/to/your/image.jpg'
with the actual path to your image. The image dimensions will be displayed next to the image in the <span>
element with the id
of imageSize
.
Note: This method retrieves the dimensions of the image after it has been rendered in the browser. If the image is not fully loaded when the function is called, the dimensions may not be accurate.
Note: You can use the method described earlier to display the size of multiple images on the same webpage.
The code to display width and height for multiple images
<!DOCTYPE html>
<html>
<head>
<script>
function showImageSize(imageId, sizeElementId) {
var img = document.getElementById(imageId);
var width = img.clientWidth;
var height = img.clientHeight;
var sizeText = width + 'px x ' + height + 'px';
var sizeElement = document.getElementById(sizeElementId);
sizeElement.textContent = sizeText;
}
</script>
</head>
<body>
<img id="image1" src="path/to/your/image1.jpg" onload="showImageSize('image1', 'imageSize1')">
<p>Image Size: <span id="imageSize1"></span></p>
<img id="image2" src="path/to/your/image2.jpg" onload="showImageSize('image2', 'imageSize2')">
<p>Image Size: <span id="imageSize2"></span></p>
<!-- Add more image elements and size elements as needed -->
</body>
</html>
we’ve modified the showImageSize
function to accept two parameters: imageId
and sizeElementId
. These parameters allow you to specify the IDs of the image element and the size element for each image individually.
When calling the function in the onload
attribute of each image, make sure to pass the corresponding image and size element IDs as arguments.
By repeating the pattern of creating image elements and size elements, you can display the size of multiple images on the same webpage.
Conclusion
Showing your users what image size they are looking at can be very useful in certain situations. This is why you learned how to display width and height of images on your website. Please tell us in the comments section if this worked for you.
Leave a Reply